Posted on 2/13/2022
Tags:
Programming,
Art
I recently needed to make pixel art for a game and wanted to define the images in code rather than having separate image asset files.
Defining the images in code comes with some benefits:
- I don't need separate versions of the images for different resolutions. I can easily scale them up automatically.
- I can easily slice the
sprites to animate them programmatically
- I can make minor tweaks with a text editor (my tool of choice! I am much more at home in a text editor than image editing software)
- I get useful diffs when changing images in
source control
To achieve this, I created a simple format I'm calling "PCEImage" (which is short for "Pixel-Character Encoded Image"). Here's what it looks like:
.:#00000000
@:#444444
#:#5555FF
.......
.@.@...
.@.@...
.@.@...
.@@@.#.
.@.@...
.@.@.#.
.@.@.#.
.@.@.#.
.......
Here's that image as a PNG (drawn with scale 10):
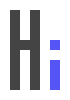
A neat benefit of this encoding is that the images are also ASCII art -- it's easy to see what a PCEImage looks like even without coloring. In fact, this format is a combination of ASCII art and
indexed color.
PCEImage Format Specification
The first section of a PCEImage defines what color each character represents. Each line in this section looks like: CHARACTER:HEXCOLOR
Then there's one blank line.
Then the second section is the image itself. Every character in this section (except for the newline at the end of each line) represents a pixel in the image. Every line in this section should be the same length (the width of the image).
There should not be a newline character after the last pixel character on the last line.
PCEImage Reference Implementation
I wrote a reference implementation of this specification in JavaScript
here.
I had a little fun and also added a wobble mode (similar to
Wobblepaint, mentioned in my
PICO-8 post).
See it in action in my
PCEImage Editor.
Read more about the editor
here.
Related:
The X PixMap (XPM) format.